INTRODUCTION
SharePoint Field Customizers are client-side components that run inside the context of a SharePoint page.
Currently, a React project is available out-of-the-box with the second option of “No JavaScript framework” for those ambitious enough to add their own preferred UI libraries.
SETUP YOUR DEVELOPMENT ENVIRONMENT
This article is not going to go into the details on how to set up your development environment. A good article can be found here.
My only recommendation is to use NVM instead of the default installation of NodeJS.
NVM allows you to install multiple versions of NodeJS and handles the heavy-lifting in swapping between them.
CREATE A FIELD CUSTOMIZER EXTENSION
As part of the article, I will demonstrate how to create a Field Customizer Extension that will display human-readable file size in Bytes, Kb, Mb, Gb, Tb, Pb, Eb, Zb, Yb from a number value store in a Sharepoint number column. In my project, I use a React component called file-bytes-formatter, but once you get the hang of it, you will be able to extend these to most React components.
Follow these steps to create the Field Customizer Extension template project. These steps have been adapted from the official Build your first Field Customizer extension article.
1) Create a new project directory in a convenient location
md Bytes-Field-Extension
2) Go to the project directory
cd Bytes-Field-Extension
3) Run the Yeoman SharePoint Generator
yo @microsoft/sharepoint
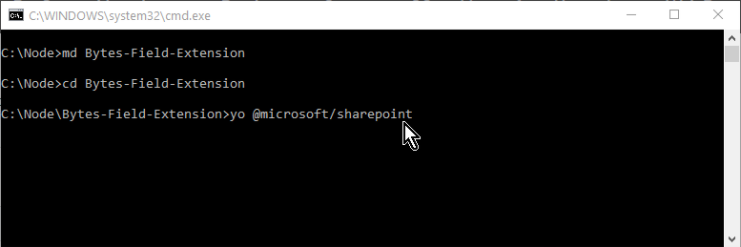
4) When prompted, enter the following values (select the default option for all prompts omitted below):
- What is your solution name?: bytes-field-extension
- Will the components in the solution require permissions to access web APIs that are unique and not shared with other components in the tenant?: (y/n) n
- Which type of client-side component to create?: Extension
- Which type of client-side extension to create?: Field Customizer
- What is your Field Customizer name?: Bytes Field Extension
- What is your Field Customizer description?: This Field Customizer Extension will return human-readable file size in Bytes, Kb, Mb, Gb, Tb, Pb, Eb, Zb, Yb
- Which framework would you like to use?: React
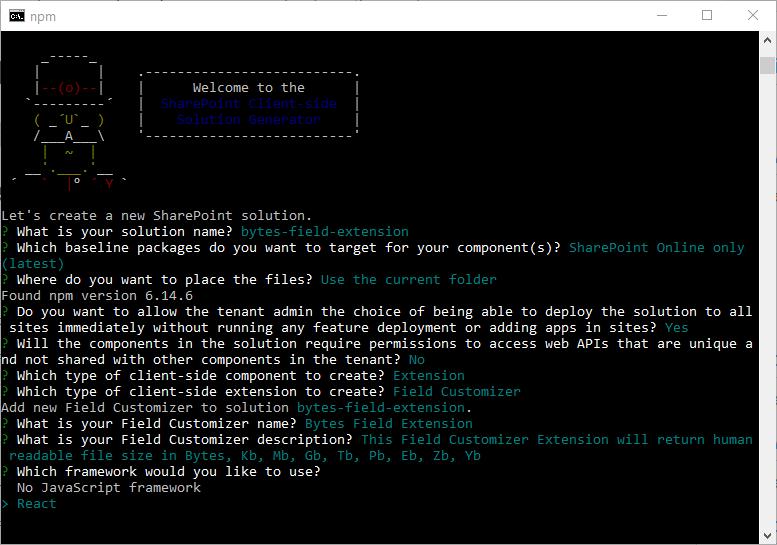
At this point, Yeoman installs the required dependencies and scaffolds the solution files along with the HelloWorld extension. This might take a few minutes. When prompted, enter the following values (select the default option for all prompts omitted below):
5) Install file-bytes-formatter React component
npm install file-bytes-formatter
6) Type the following into the console to start Visual Studio Code or open project in your favourite editor
code .
7) Within src\extensions\bytesFieldExtension\BytesFieldExtensionFieldCustomizer.ts add the following line under the last import command.
import Fsf from "file-bytes-formatter"
This will make the file-bytes-formatter component available in your project.
8) Within src\extensions\bytesFieldExtension\BytesFieldExtensionFieldCustomizer.ts change this line
const text: string = `${this.properties.sampleText}: ${event.fieldValue}`;
to
const text: string = `${Fsf(+(event.fieldValue).split(',').join(''))}`;
This will parse the text from the SharePoint column through the file-bytes-formatter component. The spit function is used to remove any commas that SharePoint might have added to style the number.
9) Make a note of the ClientSideComponentId in sharepoint\assets\elements.xml. You will need this later.
10) Save project and close project
PACKAGING FIELD CUSTOMIZER EXTENSION
1) In the console window, enter the following command to package your client-side solution that contains the extension so that we get the basic structure ready for packaging:
gulp bundle --ship
2) Execute the following command so that the solution package is created:
gulp package-solution --ship
The command creates the package: ./sharepoint/solution/bytes-field-extension.sppkg:
DEPLOYING FIELD CUSTOMIZER EXTENSION
After deploying the bytes-field-extension.sppkg into your environment (see Deploy your client-side web part to a SharePoint page if you are unfamiliar with this process) you need to configure the field that you want this formatter to take effect on.
1) Using PnP PowerShell connect to your site
Connect-PnPOnline -Url "https://YOURTENANT.sharepoint.com/sites/YOURSITE" -UseWebLogin
2) Get a reference to the field object you want to configure to use this Field Customizer Extension
$field = Get-PnPField -List "YOURLIST" -Identity "YOURFIELDNAME"
3) Set the GUID from CREATE A FIELD CUSTOMIZER EXTENSION step 9
$field.ClientSideComponentId = "YOURGUID"
4) Mark the field as updates
field.Update()
5) Execute the pending update
Invoke-PnPQuery
TEST FIELD CUSTOMIZER EXTENSION
If all sets are followed correctly any number column that has this Field Customizer Extension will take input like this.
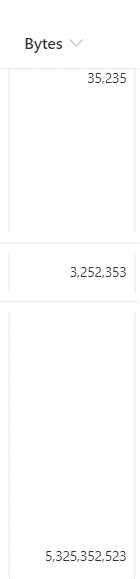
and change it to a nice human-readable format like below
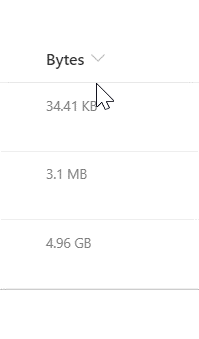
CONCLUSION
I hope you found this tutorial useful. You are encouraged to ask questions, report any bugs or make any other comments about it below.